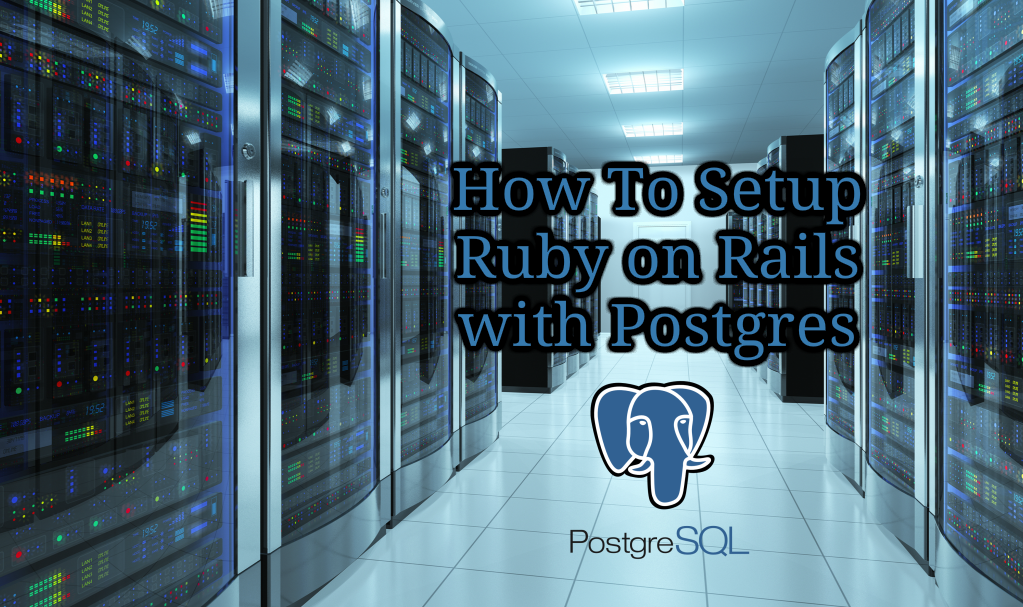
Introduction
Postgres (or PostgreSQL) is an open source database. Ruby on Rails is an open source web framework made in Ruby. Rails is database agnostic, which means that you may use it with a variety of other databases. By default, it will assume that MySQL is the database being used, however, it is also simple to use with Postgres instead.
In this tutorial we will teach you how to create a Rails application which uses a Postgres database. You may follow the tutorial on your local machine or a VPS.
Requirements
Installing Rails using RVM
The simplest way to install Rails is with RVM, this will also install Ruby.
In order to Install RVM you will have to make sure that your system has curl installed; note that these changes depending on the type of OS. If you happen to have RVM installed, feel free to skip to the next part of the tutorial.
RVM may install Ruby and Rails automatically as part of its installation. To do so, execute the following command.
curl -L https://get.rvm.io | bash -s stable --rails
Be sure to review the RVM install script before executing it, the same goes for any other remote script that you pipe into bash.
RVM is going to install itself on your system. After which, you will be able to use it to manage your Ruby versions. This is useful as you might need other versions of Ruby for various other projects. RVM also installed the Rails gem for us when we did it.
Installing Rails using RubyGems
In case you already have RVM installed, you should not have to re-install it. Instead, you may just install Rails by installing the gem.
gem install rails
This is going to install Rails and every other gem that it needs.
Installing Postgres
Please keep in mind that, when installing Postgres, it also changes depending on your OS. Look here for the whole list: postgresql.org/download.
It is simplest to use a package manager such as apt-get on Ubuntu or Homebrew on OS X.
In the case that you’re installing Postgres on a local machine, you might also need to install a GUI, however, this tutorial assumes command line usage. pgAdmin is not the best tool in the world, but it should serve its purpose here.
Finally, start installing the ‘pg’ gem so you may interface with Postgres from Ruby code. To do this, execute the below.
gem install pg
Setting Up Postgres
In this step, you’ll create a Postgres user for the Rails app. To do this, change into the Postgres user by executing the following.
su – postgres
Now we’ll create a user or a ‘role’, as Postgres says.
Create role myapp with created login password ‘password1’.
Creating Your Rails App
To make a Rails app configured for Postgres, execute the following command.
rails new myapp --database=postgresql
This is going to create a directory named ‘myapp’ which will contain an app called ‘myapp’, or you may name it as anything you’d like when running the command. Rails will expect the name of the database user to match the name of the application, however, you may change that if required.
Next, configure what database Rails will talk to. You can do this by using the ‘database.yml’ file, located at the below.
RAILS_ROOT/config/database.yml
Tip: ‘RAILS_ROOT’ is the root directory for Rails, like we’ve shown in the example above, it will be at ‘/myapp’, relative to your current location.
The ‘database.yml’ file is used by Rails to connect to the right database for the current Rails environment. It will use YAML, a data serialization standard. There are multiple databases listed here for various environments, development, production, and tests.
By default, Rails is going to assume a different database for each environment which will prove to be quite useful because, for example, the test database will be emptied and rebuilt every time you run a Rails test. For every database, make sure that the username and password match the username and password you have provided your Postgres user.
After It is configured, your ‘database.yml’ will look something like the below.
development: adapter: postgresql encoding: unicode database: myapp_development pool: 5 username: myapp password: password1 test: adapter: postgresql encoding: unicode database: myapp_test pool: 5 username: myapp password: password1
Now you can run the following.
rake db:setup
This will make development and test databases, select their owners to the user specified, and create ‘schema_migrations’ tables in each.
The table’s job is to record your migrations to schemas and data.
Running Rails
You should now be able to start your Rails app.
rails server
After navigating to ‘localhost:3000’, a Rails landing page should present itself. This won’t do much at this moment.
In order to interact with the database, create a scaffold.
rails g scaffold Post title:string body:text
rake db:migrate
Finally, go over to ‘localhost:3000/posts’, from there, you may create new posts, delete posts, and edit existing posts as you wish.
See the Rails getting started guide for more introductory operations.
Your Rails app should now be talking to a Postgres database.